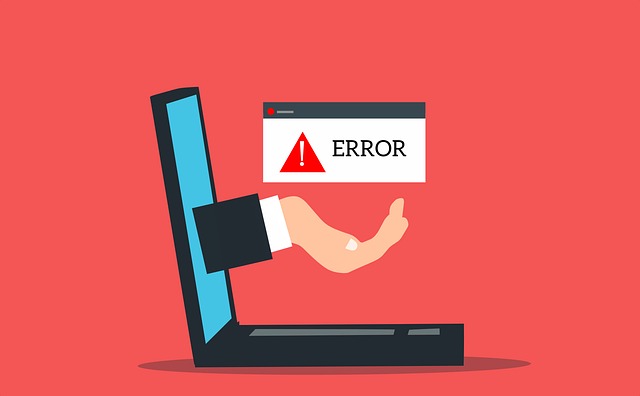
Learn how to troubleshoot and fix the “Error Call to a Member Function getCollectionParentId() on Null” in PHP applications. Understand the causes and find step-by-step solutions.
Introduction
If you’ve encountered the error call to a member function getCollectionParentId() on null while working with PHP or a PHP-based framework, you’re not alone. This error typically occurs when you’re trying to call a method on an object that hasn’t been properly instantiated, leading to a “null” reference.
Table of Contents
In this article, we’ll dive into the causes of this error, explore practical solutions, and provide best practices to help you prevent it in the future. Let’s start by understanding what this error means and why it happens.
What Does “Error Call to a Member Function getCollectionParentId() on Null” Mean?
In PHP, the error call to a member function getCollectionParentId() on null occurs when you attempt to call the getCollectionParentId()
method on a variable or object that is currently null. In other words, PHP is unable to find the object, so it throws an error instead.
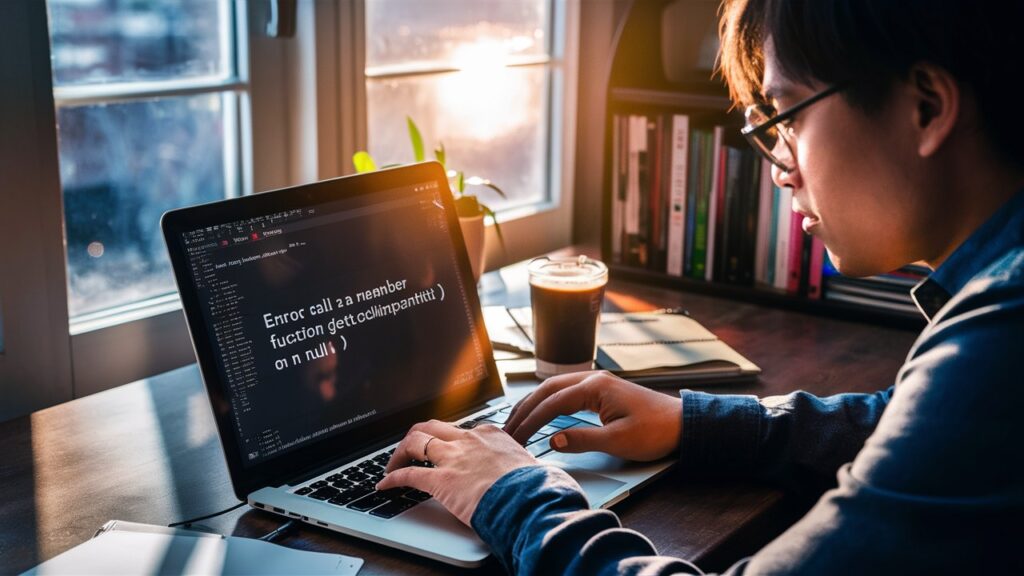
This error is common in object-oriented PHP applications, especially those that use complex data structures or relationships between objects (such as Magento, Laravel, or other frameworks that work with data collections and models).
Example Scenario:
Consider a scenario where you have the following code:
phpCopy code$parentId = $item->getCollectionParentId();
If $item
is null or not correctly instantiated as an object, PHP will throw an error stating that it can’t call getCollectionParentId()
on a null object.
Common Causes of the “Error Call to a Member Function getCollectionParentId() on Null”
To solve this error, it’s important to understand why it might be happening. Here are some of the most common causes:
- Object Not Instantiated: The variable, often an object, has not been instantiated before the method call.
- Null Value Returned from a Function: If a previous function or method returned null instead of an object, any subsequent call on that null object will trigger this error.
- Database Retrieval Issues: In frameworks that rely heavily on database objects, such as Magento or Laravel, the query might return null if the requested data is not found.
- Misconfiguration or Dependency Issues: If a function relies on external data or configurations, these might not be correctly set, causing the object to be null.
How to Fix the “Error Call to a Member Function getCollectionParentId() on Null”
Below are some approaches to help troubleshoot and resolve the error in PHP applications.
1. Check if the Object is Null Before Calling the Method
The first and simplest way to avoid this error is to check if the object is null before calling the method. You can use an if
condition to ensure that the variable is not null, as shown below:
phpCopy codeif ($item !== null) {
$parentId = $item->getCollectionParentId();
} else {
// Handle the null case, such as logging an error or setting a default value
$parentId = null;
}
By adding this check, you can prevent PHP from attempting to call a method on a null object and instead handle the null value gracefully.
2. Verify the Source of the Object
If the object is retrieved from a function or database query, check that the function is returning the expected data. For instance, if you’re using a query like find()
or get()
to fetch an item from the database, verify that it’s returning a valid object.
For example:
phpCopy code$item = $collection->getItemById($id); // Assumes getItemById() returns null if not found
if ($item) {
$parentId = $item->getCollectionParentId();
} else {
// Handle the case where no item was found
error_log("Item not found for ID: $id");
}
3. Use Dependency Injection Correctly (In Frameworks)
If you’re working in a framework like Laravel or Magento, incorrect dependency injection might lead to null values. Make sure the dependencies are correctly injected or initialized within the class, as shown below:
phpCopy codeclass SomeClass {
protected $itemRepository;
public function __construct(ItemRepository $itemRepository) {
$this->itemRepository = $itemRepository;
}
public function getParentId($id) {
$item = $this->itemRepository->find($id);
if ($item !== null) {
return $item->getCollectionParentId();
} else {
throw new Exception("Item with ID $id not found.");
}
}
}
Using dependency injection correctly helps avoid issues with uninitialized objects and reduces the risk of null values.
4. Handle Null Objects with Exception Handling
If an object is expected to be null under certain conditions, consider using a try-catch block to handle exceptions. This approach allows you to catch the error and implement fallback logic, such as logging the error for debugging purposes.
phpCopy codetry {
$parentId = $item->getCollectionParentId();
} catch (Exception $e) {
error_log("An error occurred: " . $e->getMessage());
$parentId = null; // Set a default or handle the null case
}
5. Check for Missing or Incorrect Configurations
In complex applications, missing configurations can cause objects to be null. Ensure all necessary configurations, including database connections, API keys, or other settings, are correctly set up. This is particularly crucial in frameworks where configurations are often loaded dynamically.
6. Debug with Logging
Add logging statements to track where the null object is introduced in your code. For example, log the values before and after you retrieve objects to identify when an object becomes null unexpectedly.
phpCopy codeif ($item === null) {
error_log("Item object is null. Check the data source or query.");
} else {
error_log("Item retrieved successfully: " . json_encode($item));
}
Logging provides insights into where issues might occur, helping you track down and fix bugs efficiently.
Conclusion
The error call to a member function getCollectionParentId() on null in PHP occurs when you attempt to invoke a method on a null object. While it’s a common error in PHP applications, particularly those relying on complex data structures or frameworks like Magento or Laravel, understanding the root cause is key to fixing it.
By verifying that objects are correctly initialized, using null checks, and implementing exception handling, you can effectively prevent this error. Following best practices in dependency injection, error handling, and debugging will also reduce the chances of encountering such errors in the future.
FAQs
Q1: Why do I get the “error call to a member function getCollectionParentId() on null”?
- This error occurs when PHP tries to call
getCollectionParentId()
on an object that is null. This can happen if the object wasn’t properly initialized or if a query didn’t return the expected result.
Q2: How can I prevent this error from occurring?
- Always check if an object is null before calling methods on it. Use conditional checks, exception handling, and ensure your code has no missing dependencies or misconfigurations.
Q3: What should I do if the object is still null after applying fixes?
- Double-check the source of the object, especially if it’s retrieved from a database or an external API. Debug by logging the object values before calling methods to understand where the null value originates.
Q4: Are there specific PHP frameworks where this error is more common?
- Yes, frameworks like Magento, Laravel, and Symfony, which frequently deal with object-relational mapping (ORM) and complex data structures, often experience this type of error.
Q5: Can dependency injection help in resolving null reference errors?
- Yes, using dependency injection ensures that all required dependencies are properly initialized, reducing the chances of encountering null reference errors.
Handling errors like “call to a member function getCollectionParentId() on null” proactively can improve your application’s stability and enhance your debugging skills in PHP. By following these guidelines, you’ll be better equipped to prevent and resolve null reference issues in your code.